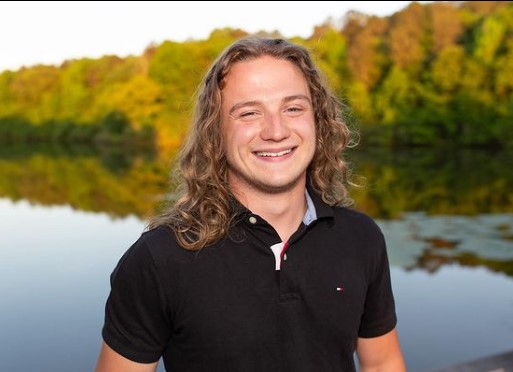
Welcome to My Portfolio
I recently earned an associate's degree in computer programming, and I am seeking an entry-level position where I can apply the skills and knowledge I've gained during my studies.
From a young age, I have been immersed in the world of computers, receiving my first personal computer at the age of seven. This early exposure sparked a lifelong journey of studying how computers work and honing my skills in using them proficiently.
Explore my projects and see what I’ve been working on:
Projects
Warehouse Inventory Management System
This project involves developing a JavaFX application for warehouse inventory management, using Apache POI for Excel file handling. The application allows users to input item details, select images for packing lists and damaged items, and create new spreadsheet reports.
Plots for Price
Plots for Price is a Java program that allows users to navigate over a plot of land from an image. When they hover over a certain plot, it displays the price of that plot.
import java.util.Scanner;
import java.awt.*; // classes for building GUI components
import javax.swing.*; // GUI components for building desktop applications
import java.awt.event.*; // classes for handling events that occur in GUI components
import java.io.*; // classes for reading and writing data to files and other sources
import java.text.DecimalFormat; // classes for formatting and parsing numbers and dates
import java.util.ArrayList; // used to store the boundaries of the plot, along with their names and prices
public class PlotPriceCalculator extends JFrame implements MouseMotionListener {
private Image image; // private member variable that holds the loaded image
private ArrayList boundaries; // private member variable that holds the boundaries of each plot
private JLabel priceLabel; // private member variable that holds the price of the plot
private JLabel pricePerSqftLabel; // private member variable that holds price per square feet labels
private DecimalFormat df; // df is a DecimalFormat object that formats the price per square feet to two decimal places
// constructor for the PlotPriceCalculator class that sets up the JFrame with a title, a fixed size, and a border layout
public PlotPriceCalculator() {
setTitle("Company X Plot Price Calculator");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setResizable(false);
setSize(630, 640);
setLocationRelativeTo(null);
setLayout(new BorderLayout());
// loads the image of the plots from a file and catches any exception that occurs during the loading process
try {
image = new ImageIcon("src/image/PlotsByPrice.jpg").getImage();
} catch (Exception error) {
System.out.println("Can't load the image: " + error.getMessage());
System.exit(1);
}
// creating a new JPanel and overriding the paintComponent method to draw the loaded image on it
JPanel imagePanel = new JPanel() {
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.drawImage(image, 0, 0, null);
}
};
// the panel's preferred size is set to the size of the image, and a MouseMotionListener is added to the panel to listen for mouse movement events
imagePanel.setPreferredSize(new Dimension(image.getWidth(null), image.getHeight(null)));
imagePanel.addMouseMotionListener(this);
add(imagePanel, BorderLayout.CENTER);
// loading the boundaries of each plot from the data file and storing them in an ArrayList of Boundary objects
boundaries = new ArrayList<>();
df = new DecimalFormat("#.##"); // DecimalFormat object to format the price per square feet
try {
Scanner input = new Scanner(new File("src/data/CompanyX.dat"));
while (input.hasNext()) {
String name = input.nextLine();
String[] c = input.nextLine().split("\\s+");
int[][] points = new int[c.length / 2][2];
for (int i = 0; i < c.length; i += 2) {
points[i / 2][0] = Integer.parseInt(c[i]);
points[i / 2][1] = Integer.parseInt(c[i + 1]);
}
double price = Double.parseDouble(input.nextLine());
boundaries.add(new Boundary(name, points, price));
}
input.close();
} catch (Exception error) {
System.out.println("Can't load data file: " + error.getMessage());
System.exit(1);
}
// add a panel to show the price and price per square feet of the plot
JPanel infoPanel = new JPanel(new GridLayout(2, 2));
infoPanel.setBorder(BorderFactory.createLineBorder(Color.BLACK));
JLabel priceTextLabel = new JLabel("Price: ");
priceLabel = new JLabel();
JLabel pricePerSqftTextLabel = new JLabel("Price per sqft: ");
pricePerSqftLabel = new JLabel();
infoPanel.add(priceTextLabel);
infoPanel.add(priceLabel);
infoPanel.add(pricePerSqftTextLabel);
infoPanel.add(pricePerSqftLabel);
add(infoPanel, BorderLayout.SOUTH);
}
public static void main(String[] args) {
// making the PlotPriceCalculator visible
new PlotPriceCalculator().setVisible(true);
}
@Override
public void mouseMoved(MouseEvent e) {
// variables to keep track of whether the mouse is inside a boundary and which boundary is selected
boolean insideBoundary = false;
Boundary selectedBoundary = null;
// loop through all boundaries to check if the mouse is inside one of them
for (Boundary boundary : boundaries) {
if (boundary.contains(e.getX(), e.getY())) {
insideBoundary = true;
selectedBoundary = boundary;
break;
}
}
// if the mouse is inside a boundary, update the price and pricePerSqft labels with the corresponding values
if (insideBoundary) {
priceLabel.setText("$" + selectedBoundary.getPrice());
double area = selectedBoundary.getArea();
double pricePerSqft = selectedBoundary.getPrice() / area;
pricePerSqftLabel.setText("$" + df.format(pricePerSqft) + " per sqft");
} else {
// if the mouse is not inside a boundary, clear the price and pricePerSqft labels
priceLabel.setText("");
pricePerSqftLabel.setText("");
}
}
@Override
public void mouseDragged(MouseEvent e) {
// required to use MouseMotionListener
}
}
class Boundary {
private String name;
private int[][] points;
private double price;
public Boundary(String name, int[][] points, double price) {
// constructor
this.name = name;
this.points = points;
this.price = price;
}
public String getName() {
// getter method
return name;
}
public int[][] getPoints() {
// getter method
return points;
}
// getter method
public double getPrice() {
return price;
}
// calculate the area of the boundary using the shoelace formula
public double getArea() {
double area = 0.0;
int j = points.length - 1;
for (int i = 0; i < points.length; i++) {
area += (points[j][0] + points[i][0]) * (points[j][1] - points[i][1]);
j = i;
}
return Math.abs(area / 2.0);
}
// check if a point (x, y) is inside the boundary using the ray casting algorithm
public boolean contains(int x, int y) {
int i;
int j;
boolean result = false;
for (i = 0, j = points.length - 1; i < points.length; j = i++) {
if ((points[i][1] > y) != (points[j][1] > y) &&
(x < (points[j][0] - points[i][0]) * (y - points[i][1]) / (points[j][1] - points[i][1]) + points[i][0])) {
result = !result;
}
}
return result;
}
}
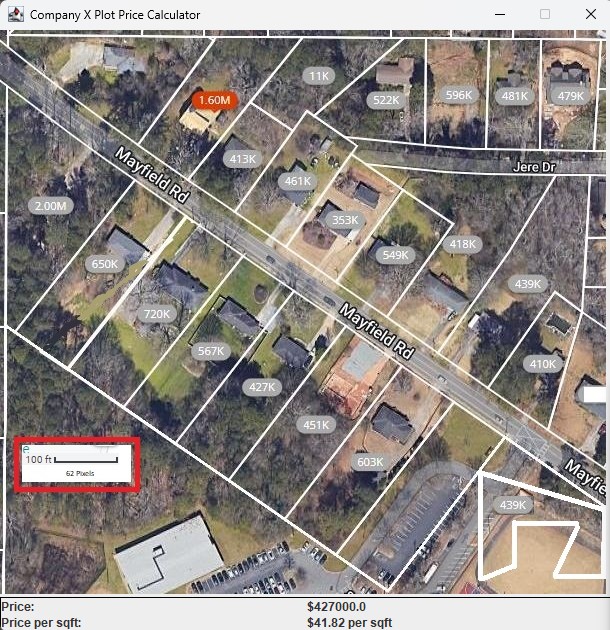
Daily Calorie Tracker
This Python project helps users track their daily calorie intake. It provides a menu with options to add or remove calories, restart the day, end the day, and exit the program. The program also stores the total calories for each day in a file.
calories = [] # list to store the calories
# function to add calories to the list
def addCalories():
try:
calories.append(int(input("Enter the number of calories: ")))
except ValueError: # if the user enters anything other than a number
print("Invalid input")
# function to remove calories from the list
def removeCalories():
try:
calories.remove(int(input("Enter the number of calories: ")))
except ValueError: # if the user enters anything other than a number in the calories list
print("Calories not found in list.")
# function to restart the day by clearing the calories list
def restartDay():
calories.clear()
print("Calories list cleared.")
# function to end the day by calculating and storing the total calories to a file
def endDay(day):
totalCalories = sum(calories)
print("Total calories for day", day, ":", totalCalories)
storeFile(day, totalCalories)
calories.clear() # clearing the calories list after storing the calories in the file
# function to store the total calories to a file
def storeFile(day, totalCalories):
try:
with open("calories.txt", "a") as f: # using "with" statement to automatically close the file
f.write("Day " + str(day) + ": " + str(totalCalories) + "\n") # writing the total calories to the file
print("Calories written to file.")
except FileNotFoundError:
print("File not found.")
except Exception as e:
print("Error occurred while writing to file:", str(e))
# function to display the menu
def menu():
print("-----------------------------------")
print("1. Add calories for the day.")
print("2. Remove calories for the day.")
print("3. Restart the day.")
print("4. End the day.")
print("5. Exit.")
print("-----------------------------------")
# main function to run the program
def main():
day = 1 # start with day 1
while True:
menu() # displaying the menu
choice = input("Enter your choice: ") # getting user input for choice
if choice == "1":
addCalories() # calling addCalories function if choice is 1
elif choice == "2":
removeCalories() # calling removeCalories function if choice is 2
elif choice == "3":
restartDay() # calling restartDay function if choice is 3
elif choice == "4":
endDay(day) # calling endDay function if choice is 4
day += 1 # increment day
elif choice == "5":
break # breaking the loop and exiting the program
else:
print("Invalid choice.")
if __name__ == "__main__": # ensure that the code inside the block is only executed when the script is run directly
main()
Retail Sales Management System
The Retail Sales Management System was developed using Java. It includes features for managing retail items, processing sales transactions, and generating sales receipts. The system allows for adding and updating item details, calculating subtotal, sales tax, and total for purchases, and creating detailed sales receipts for record-keeping.
User Account Management System
This C++ project is a user account management system that allows for creating, logging in, changing passwords, and deleting accounts. The program begins by asking the user how many accounts to create and enforces specific password requirements. Users can then log into their accounts, change passwords, and delete accounts using a menu-driven interface. The system ensures security by verifying usernames and passwords and provides appropriate messages for incorrect login attempts.
/******************************************************************************
Basic Template for our C++ Programs.
*******************************************************************************/
#include /* printf, scanf, puts, NULL */
#include /* srand, rand */
#include /* time */
#include // String managment funtions.
#include // For input and output
#include // For math functions.
#include
#include
#include
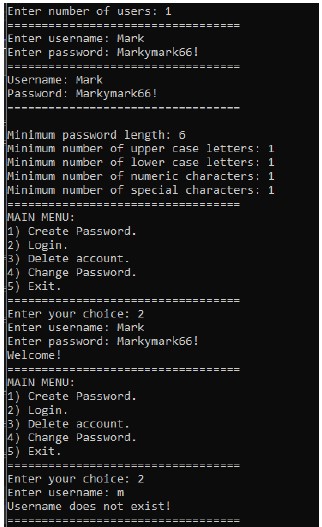
Contact
If you would like to get in touch, please reach out to me via email at markymark777.2115@yahoo.com.